What is Function Overiding Problem in C++ and how to Solve?
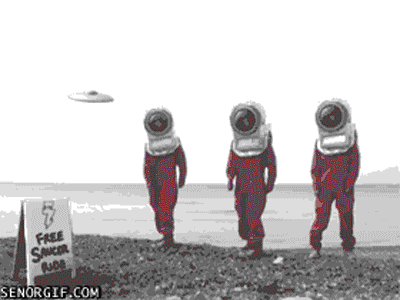
First Let Us Understand what is function overriding problem!
Inheritance in C++ Inheritance allows software developers to derive a new class from the existing class. The derived class inherits features of the base class (existing class).
C++ allows you to specify more than one definition for a function name or an operator in the same scope, which is called function overloading and operator overloadingrespectively.
An overloaded declaration is a declaration that is declared with the same name as a previously declared declaration in the same scope, except that both declarations have different arguments and obviously different definition (implementation).
This Causes Chaos and Confusion !!
Lets Get a Wide Perspective By Seeing this
Code of Function Overiding
This Is a Typical Function Overriding Program:-
As u can see when we call the above function the compiler will get confused as to which function to call.
See sum value in output .
`*sum=4354132* <——- This Is a Random Garbage Value.
So How Do i Solve it???
Dont Worry Sailor i Got Your Back Covered!!
Observe This Code:-
Got A Eureka Sensation!!
Its That easy!!
Here is a Program to Keep U started
#include <iostream>
using namespace std;
class Base
{
public:
int a,b;
void getData(){
cout<<"Enter values of a & b:";
cin>>a>>b;
}
};
class Derived:public Base
{
public:
int sum;
void getData(){
Base::getData();
sum=a+b;
cout<<endl<<"sum="<<sum;
}
};
int main()
{
Derived obj;
obj.getData();
}
Enter values of a & b: 10 20 sum=30